Compute Total Number of Distinct Ways to Reach Target
Get started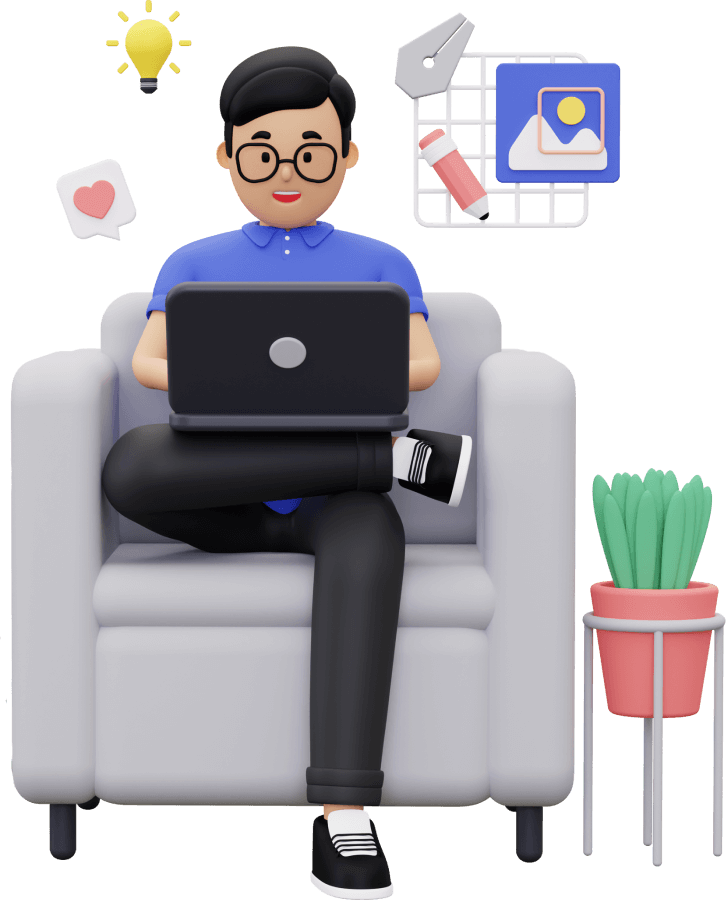
জয় শ্রী রাম
🕉The DP problems belonging to this category, in its simplest form, looks like below or some variation of it:
- Given a target find a number of distinct ways to reach the target.
The solution for this kind of problems, in a generalized form, would often look like below:
-
Sum all possible ways to reach the current state.
distinctWays[i] = distinctWays[i-1] + distinctWays[i-2] + ... + distinctWays[i-k]
, where (i - 1), (i - 2), ... , (i - K) are all the directly immediate states from where i can be reached. -
Overall the solution would look like:
for (int curr = 1; curr <= target; curr++) { for (int k = 0; k < waysToReachCurrentTarget.size(); k++) { dp[i] += dp[waysToReachCurrentTarget[k]]; } } return dp[target];
The below problem would give you a basic idea about this kind of problems:
Problem Statement:
You are climbing a stair case. It takes n steps to reach to the top.
Each time you can either climb 1 or 2 steps. In how many distinct ways can you climb to the top?
Example 1:
Input: 2
Output: 2
Explanation: There are two ways to climb to the top.
1. 1 step + 1 step
2. 2 steps
Example 2:
Input: 3
Output: 3
Explanation: There are three ways to climb to the top.
1. 1 step + 1 step + 1 step
2. 1 step + 2 steps
3. 2 steps + 1 step
Solution in Java and Python:
Login to Access Content