T9 Keyboard
Get started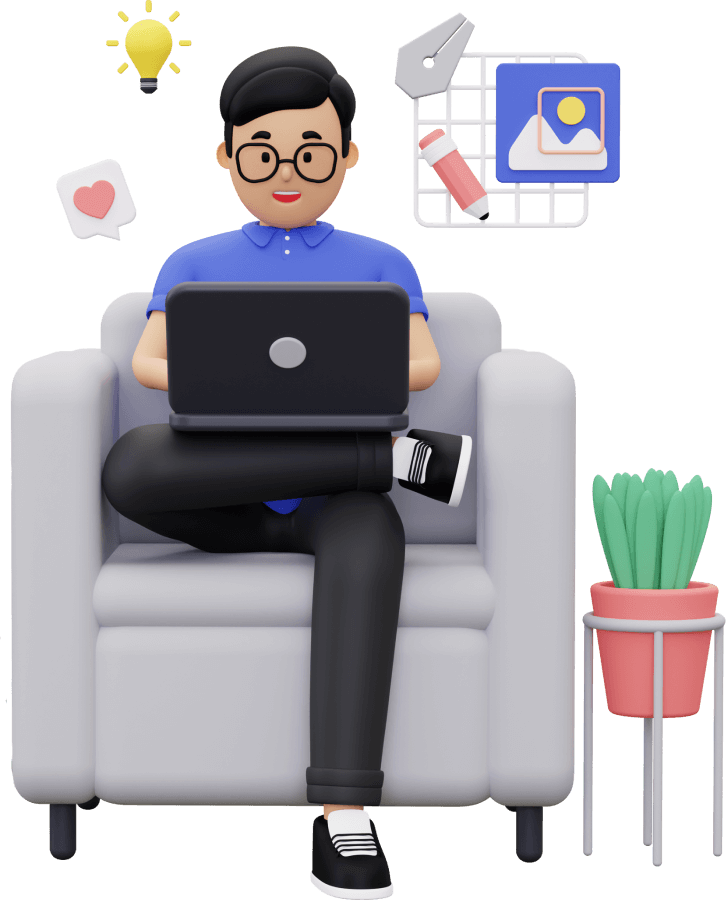
জয় শ্রী রাম
🕉Problem Statement:
Given a string containing digits from 2-9 inclusive, return all possible letter combinations that the number could represent. Return the answer in any order.
A mapping of digit to letters (just like on the telephone buttons) is given below. Note that 1 does not map to any letters.
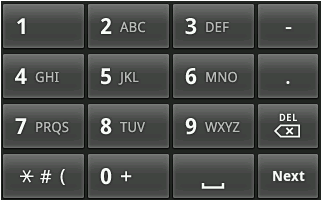
Example 1:
Input: digits = "23"
Output: ["ad","ae","af","bd","be","bf","cd","ce","cf"]
Example 2:
Input: digits = ""
Output: []
Example 3:
Input: digits = "2"
Output: ["a","b","c"]
Solution:
- NOTE: I highly recommend going through the Backtracking chapters in the order they are given in the Index page to get the most out of it and be able to build a rock-solid understanding.
You need to get yourself familiar with Backtracking Template before going through the solution, if you haven't done that already.
Let's say digits[0...(n-1)] is the given phone number.
We can solve this problem by doing an exhaustive search.
We start from digits[i] where i = 0.
Candidates for the i-th character of the solution would all the possible letters for digits[i]. For each of these candidates, candidates for (i + 1)-th character of the solution would all possible letters for digits[i + 1]. We go on doing this till we have processed for the last character of the solutions.
What we just described above is nothing but Backtracking. Using our backtracking template we get the below code:
Java Code:
Login to Access Content
Python Code:
Login to Access Content
Don't forget to take in-depth look at the below problems because that is what would make you comfortable with using the backtracking template and master the art of Backtracking:
- Letter Case Permutation
- Power Set
- All Paths Between Two Nodes
- Word Search
- Sudoku
- N-Queens
- Word Square
- Generate Parentheses